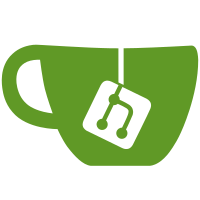
Se requiere mas verificacion y respaldar el registro, ademas de chequear que no se puedan registrar mas de una vez. Mandar un mail para confirmarle al usuario que se registró ok. Agregar el captcha al formulario de contacto y las verificaciones faltantes
141 lines
3.9 KiB
PHP
141 lines
3.9 KiB
PHP
<?php
|
|
|
|
function verifyCaptcha(){
|
|
$url = 'https://www.google.com/recaptcha/api/siteverify';
|
|
$data = array(
|
|
'secret' => '6LeLxy4UAAAAABClplWLJUjZ1_nhX_-SI7CuNcm8',
|
|
'response' => $_POST["g-recaptcha-response"]
|
|
);
|
|
$options = array(
|
|
'http' => array (
|
|
'header' => 'Content-Type: application/x-www-form-urlencoded\r\n',
|
|
'method' => 'POST',
|
|
'content' => http_build_query($data)
|
|
)
|
|
);
|
|
$context = stream_context_create($options);
|
|
$verify = file_get_contents($url, false, $context);
|
|
$captcha_success=json_decode($verify);
|
|
return $captcha_success;
|
|
}
|
|
|
|
function setheaders() {
|
|
$fp = fopen("2021y22.csv", 'w');
|
|
$cabezal = array('Nombre', 'Apellido','TipoDoc','Documento',
|
|
'Direccion','Pais','Ciudad', 'Telefono', 'Email',
|
|
'Profesión','Trabaja en','Financiación','Detalle Financiación');
|
|
if($fp){
|
|
fputcsv($fp,$cabezal);
|
|
fclose($fp);
|
|
}
|
|
else{
|
|
die("unable to open file");
|
|
}
|
|
}
|
|
|
|
function registrar($fila) {
|
|
$fp = fopen("2021y22.csv", 'a');
|
|
if($fp){
|
|
fputcsv($fp,$fila);
|
|
fclose($fp);
|
|
|
|
}
|
|
else{
|
|
die("unable to open file");
|
|
}
|
|
|
|
}
|
|
|
|
$errors = array(); // array to hold validation errors
|
|
$data = array(); // array to pass back data
|
|
$filename = "2021y22";
|
|
|
|
// validate the variables ======================================================
|
|
$data['nombre'] = $_POST['nombre'];
|
|
$data['apellido'] = $_POST['apellido'];
|
|
$data['tipodoc'] = $_POST['doctype'];
|
|
$data['nrodoc'] = $_POST['docnro'];
|
|
$data['direccion'] = $_POST['dir'];
|
|
$data['pais'] = $_POST['pais'];
|
|
$data['ciudad'] = $_POST['ciudad'];
|
|
$data['telefono'] = $_POST['tel'];
|
|
$data['email'] = $_POST['email'];
|
|
$data['profesion'] = $_POST['profesion'];
|
|
$data['trabaja'] = $_POST['trabaja'];
|
|
$data['financiacion'] = $_POST['financiacion'];
|
|
$data['detallefinan'] = $_POST['detallefinan'];
|
|
|
|
if (empty($_POST['nombre']))
|
|
$errors['nombre'] = 'Nombre is required.';
|
|
|
|
if (empty($_POST['apellido']))
|
|
$errors['apellido'] = 'Apellido is required.';
|
|
|
|
if (empty($_POST['doctype']))
|
|
$errors['doctype'] = 'No seleccionó un tipo de doc';
|
|
|
|
if (empty($_POST['docnro']))
|
|
$errors['docnro'] = 'No ingreso un documento';
|
|
|
|
if (empty($_POST['dir']))
|
|
$errors['dir'] = 'No ingreso una direccion';
|
|
|
|
if (empty($_POST['pais']))
|
|
$errors['pais'] = 'Ingrese el pais de presedencia';
|
|
|
|
if (empty($_POST['ciudad']))
|
|
$errors['ciudad'] = 'Ingrese ciudad de presedencia';
|
|
|
|
if (empty($_POST['tel']))
|
|
$errors['ciudad'] = 'Telefono de contacto vacio o incorrecto';
|
|
|
|
if (empty($_POST['email']))
|
|
$errors['email'] = 'E-Mail de contacto vacio o incorrecto';
|
|
|
|
if (empty($_POST['profesion']))
|
|
$errors['email'] = 'indique profesion';
|
|
|
|
if (empty($_POST['trabaja']))
|
|
$errors['trabaja'] = 'Indique en el sector que trabaja';
|
|
if(empty($_POST['g-recaptcha-response'])){
|
|
$errors['recaptcha'] = 'Debe validar el captcha';
|
|
}
|
|
else if(!verifyCaptcha()){
|
|
$errors['recaptcha'] = 'Error en la validación de ReCaptcha';
|
|
}
|
|
|
|
|
|
/*if ($_POST['g-recaptcha-response']){
|
|
$response = $reCaptcha->verifyResponse(
|
|
$_SERVER["REMOTE_ADDR"],
|
|
$_POST["g-recaptcha-response"]
|
|
);
|
|
if($response == null || !$response->sucess)
|
|
$errors['captcha'] = 'no captcha seleceted';
|
|
}
|
|
else{
|
|
echo "falta el captcha";
|
|
}*/
|
|
|
|
if( !file_exists("2021y22.csv"))
|
|
setheaders();
|
|
|
|
// return a response ===========================================================
|
|
// if there are any errors in our errors array, return a success boolean of false
|
|
if ( ! empty($errors)) {
|
|
|
|
// if there are items in our errors array, return those errors
|
|
$data['success'] = false;
|
|
$data['errors'] = $errors;
|
|
}
|
|
else {
|
|
$fila = $data;
|
|
registrar($fila);
|
|
$data['success'] = true;
|
|
$data['message'] = 'Registro exitoso';
|
|
}
|
|
|
|
// return all our data to an AJAX call
|
|
echo json_encode($data);
|
|
|
|
?>
|